Question 1 :
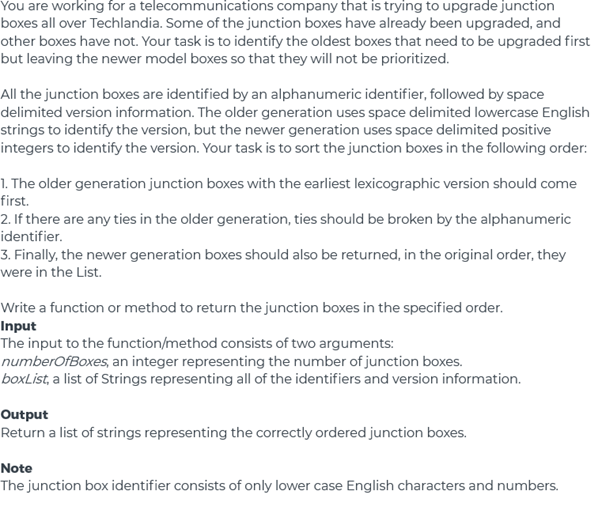
Below gives the expected results for the used sample -
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
public class Techlandia {
static HashMap<String, List<String>> temp = new HashMap<>();
static ArrayList<String> values = new ArrayList<>();
static ArrayList<String> news = new ArrayList<>();
static HashMap<String, String> olds = new HashMap<>();
static List<String> results = new ArrayList<>();
public static void main(String[] args) {
List<String> boxList = new ArrayList<>();
boxList.add("ykc 82 01");
boxList.add("eo first qpx");
boxList.add("09z cat hamster");
boxList.add("06f 12 25 6");
boxList.add("az0 first qpx");
boxList.add("236 cat dog rabbit snake");
orderedJunctionBoxes(6, boxList).forEach(System.out::println);
}
public static List<String> orderedJunctionBoxes(int numberOfBoxes,
List<String> boxList) {
boxList.forEach(a -> {
int p = a.indexOf(" ");
String version = a.substring(p+1);
String id = a.substring(0, p);
String[] vSplit = version.split(" ");
try {
Integer.parseInt(vSplit[0]);
news.add(a);
} catch(NumberFormatException nfe) {
List<String> ls;
if((ls=temp.get(version)) == null) {
ls = new ArrayList<>();
ls.add(id);
temp.put(version, ls);
} else {
ls.add(id);
temp.put(version, ls);
}
olds.put(id, a);
values.add(version);
}
});
Collections.sort(values);
values.forEach(a -> {
List<String> vals = temp.get(a);
Collections.sort(vals);
vals.forEach(x -> {
results.add(olds.get(x));
});
temp.put(a, new ArrayList<String>());
});
news.forEach(a -> results.add(a));
return results;
}
}
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
public class Techlandia {
static HashMap<String, List<String>> temp = new HashMap<>();
static ArrayList<String> values = new ArrayList<>();
static ArrayList<String> news = new ArrayList<>();
static HashMap<String, String> olds = new HashMap<>();
static List<String> results = new ArrayList<>();
public static void main(String[] args) {
List<String> boxList = new ArrayList<>();
boxList.add("ykc 82 01");
boxList.add("eo first qpx");
boxList.add("09z cat hamster");
boxList.add("06f 12 25 6");
boxList.add("az0 first qpx");
boxList.add("236 cat dog rabbit snake");
orderedJunctionBoxes(6, boxList).forEach(System.out::println);
}
public static List<String> orderedJunctionBoxes(int numberOfBoxes,
List<String> boxList) {
boxList.forEach(a -> {
int p = a.indexOf(" ");
String version = a.substring(p+1);
String id = a.substring(0, p);
String[] vSplit = version.split(" ");
try {
Integer.parseInt(vSplit[0]);
news.add(a);
} catch(NumberFormatException nfe) {
List<String> ls;
if((ls=temp.get(version)) == null) {
ls = new ArrayList<>();
ls.add(id);
temp.put(version, ls);
} else {
ls.add(id);
temp.put(version, ls);
}
olds.put(id, a);
values.add(version);
}
});
Collections.sort(values);
values.forEach(a -> {
List<String> vals = temp.get(a);
Collections.sort(vals);
vals.forEach(x -> {
results.add(olds.get(x));
});
temp.put(a, new ArrayList<String>());
});
news.forEach(a -> results.add(a));
return results;
}
}
Question 2 :
Below gives the expected results for the used sample -
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
public class Apps {
public static void main(String[] args) {
List<List<Integer>> foregroundAppList = new ArrayList<>();
List<Integer> temp = new ArrayList<>();
temp.add(1);
temp.add(3);
foregroundAppList.add(temp);
temp = new ArrayList<>();
temp.add(2);
temp.add(5);
foregroundAppList.add(temp);
temp = new ArrayList<>();
temp.add(3);
temp.add(7);
foregroundAppList.add(temp);
temp = new ArrayList<>();
temp.add(4);
temp.add(10);
foregroundAppList.add(temp);
List<List<Integer>> backgroundAppList = new ArrayList<>();
temp = new ArrayList<>();
temp.add(1);
temp.add(2);
backgroundAppList.add(temp);
temp = new ArrayList<>();
temp.add(2);
temp.add(3);
backgroundAppList.add(temp);
temp = new ArrayList<>();
temp.add(3);
temp.add(4);
backgroundAppList.add(temp);
temp = new ArrayList<>();
temp.add(4);
temp.add(5);
backgroundAppList.add(temp);
optimalUtilization(7, foregroundAppList, backgroundAppList).forEach(System.out::println);
}
static List<List<Integer>> optimalUtilization(int deviceCapacity,
List<List<Integer>> foregroundAppList,
List<List<Integer>> backgroundAppList) {
List<Integer> foregroundCap = new ArrayList<>();
List<Integer> backgroundCap = new ArrayList<>();
HashMap<Integer, Integer> foreCaps = new HashMap<>();
HashMap<Integer, Integer> backCaps = new HashMap<>();
int tempV;
int max = 0;
foregroundAppList.forEach(a -> {
foregroundCap.add(a.get(1));
foreCaps.put(a.get(1), a.get(0));
});
backgroundAppList.forEach(a ->{
backgroundCap.add(a.get(1));
backCaps.put(a.get(1), a.get(0));
});
Collections.sort(backgroundCap);
int bl = backgroundCap.size();
Collections.sort(foregroundCap);
int fl = foregroundCap.size();
int k = fl < bl ? fl : bl;
List<List<Integer>> results = new ArrayList<>(k);
for(int x = fl-1; x >= 0; x--) {
int a = foregroundCap.get(x);
int rem = deviceCapacity - a;
if(rem <= 0)
continue;
if(backCaps.containsKey(rem)) {
List<Integer> temp = new ArrayList<>();
temp.add(foreCaps.get(a));
temp.add(backCaps.get(rem));
if(results.isEmpty())
results.add(temp);
else
results.add(results.size()-1,temp);
} else {
for(int i = bl-1; i >= 0 && results.isEmpty(); i--) {
if(rem-backgroundCap.get(i) > max) {
tempV = backgroundCap.get(i);
List<Integer> temp = new ArrayList<>();
temp.add(foreCaps.get(a));
temp.add(backCaps.get(tempV));
results.add(temp);
max = a + tempV;
break;
}
}
}
}
return results;
}
}
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
public class Apps {
public static void main(String[] args) {
List<List<Integer>> foregroundAppList = new ArrayList<>();
List<Integer> temp = new ArrayList<>();
temp.add(1);
temp.add(3);
foregroundAppList.add(temp);
temp = new ArrayList<>();
temp.add(2);
temp.add(5);
foregroundAppList.add(temp);
temp = new ArrayList<>();
temp.add(3);
temp.add(7);
foregroundAppList.add(temp);
temp = new ArrayList<>();
temp.add(4);
temp.add(10);
foregroundAppList.add(temp);
List<List<Integer>> backgroundAppList = new ArrayList<>();
temp = new ArrayList<>();
temp.add(1);
temp.add(2);
backgroundAppList.add(temp);
temp = new ArrayList<>();
temp.add(2);
temp.add(3);
backgroundAppList.add(temp);
temp = new ArrayList<>();
temp.add(3);
temp.add(4);
backgroundAppList.add(temp);
temp = new ArrayList<>();
temp.add(4);
temp.add(5);
backgroundAppList.add(temp);
optimalUtilization(7, foregroundAppList, backgroundAppList).forEach(System.out::println);
}
static List<List<Integer>> optimalUtilization(int deviceCapacity,
List<List<Integer>> foregroundAppList,
List<List<Integer>> backgroundAppList) {
List<Integer> foregroundCap = new ArrayList<>();
List<Integer> backgroundCap = new ArrayList<>();
HashMap<Integer, Integer> foreCaps = new HashMap<>();
HashMap<Integer, Integer> backCaps = new HashMap<>();
int tempV;
int max = 0;
foregroundAppList.forEach(a -> {
foregroundCap.add(a.get(1));
foreCaps.put(a.get(1), a.get(0));
});
backgroundAppList.forEach(a ->{
backgroundCap.add(a.get(1));
backCaps.put(a.get(1), a.get(0));
});
Collections.sort(backgroundCap);
int bl = backgroundCap.size();
Collections.sort(foregroundCap);
int fl = foregroundCap.size();
int k = fl < bl ? fl : bl;
List<List<Integer>> results = new ArrayList<>(k);
for(int x = fl-1; x >= 0; x--) {
int a = foregroundCap.get(x);
int rem = deviceCapacity - a;
if(rem <= 0)
continue;
if(backCaps.containsKey(rem)) {
List<Integer> temp = new ArrayList<>();
temp.add(foreCaps.get(a));
temp.add(backCaps.get(rem));
if(results.isEmpty())
results.add(temp);
else
results.add(results.size()-1,temp);
} else {
for(int i = bl-1; i >= 0 && results.isEmpty(); i--) {
if(rem-backgroundCap.get(i) > max) {
tempV = backgroundCap.get(i);
List<Integer> temp = new ArrayList<>();
temp.add(foreCaps.get(a));
temp.add(backCaps.get(tempV));
results.add(temp);
max = a + tempV;
break;
}
}
}
}
return results;
}
}